[SOLVED] Python ValueError | Causes and Solutions
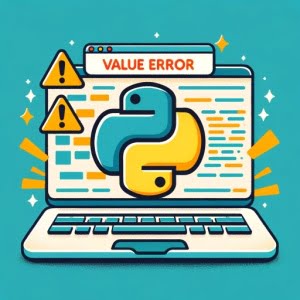
Are you finding it challenging to understand or resolve a ValueError in Python? You’re not alone. Many developers, especially those new to Python, often find themselves puzzled when they encounter a ValueError in their code.
Think of a ValueError as a traffic signal in your code – it stops the flow of your program when it encounters an inappropriate value. It’s a mechanism Python uses to ensure that the functions and operations in your code are receiving the right kind of values they expect.
This guide will help you understand what a ValueError is, why it occurs, and how to effectively handle it in your Python programs. We’ll cover everything from the basics of ValueError, its causes, to more advanced techniques of handling and resolving it, as well as alternative approaches.
So, let’s dive in and start mastering ValueError in Python!
TL;DR: What is a ValueError in Python and How Do I Handle It?
A ValueError in Python is raised when a function receives an argument of the correct type but an inappropriate value. To handle it, you can use a try-except block to catch the error and handle it appropriately.
Here’s a simple example:
def square_root(n):
if n < 0:
raise ValueError('n must be a non-negative number')
return n ** 0.5
try:
print(square_root(-1))
except ValueError as e:
print(e)
# Output:
# 'n must be a non-negative number'
In this example, we define a function square_root
that calculates the square root of a number. However, it raises a ValueError if the input is a negative number. We then use a try-except block to catch this ValueError when we try to calculate the square root of -1.
This is a basic way to handle ValueError in Python, but there’s much more to learn about error handling in Python. Continue reading for a more detailed discussion on ValueError and how to handle it.
Table of Contents
- Unraveling ValueError: A Beginner’s Guide
- Tackling Complex Scenarios: ValueError
- Exploring Alternate Error Handling Techniques
- Troubleshooting ValueError: Avoiding Common Pitfalls
- Python Exception Handling: Understanding ValueError
- Beyond ValueError: Building Robust Python Programs
- Wrapping Up: Mastering ValueError in Python
Unraveling ValueError: A Beginner’s Guide
A ValueError in Python is a type of exception that occurs when a function receives an argument of the correct type but an inappropriate value. This error is often encountered when you’re trying to perform an operation that requires a certain kind of value but you’re providing a value that doesn’t fit the bill.
Let’s take an example to understand this better:
# A simple function to convert a string into an integer
def convert_to_int(string):
return int(string)
try:
print(convert_to_int('123'))
print(convert_to_int('abc'))
except ValueError as e:
print(f'Caught a ValueError: {e}')
# Output:
# 123
# Caught a ValueError: invalid literal for int() with base 10: 'abc'
In the above example, the function convert_to_int
is designed to convert a string into an integer. It works perfectly fine when we pass ‘123’ (a string that can be converted to an integer). However, when we pass ‘abc’ (a string that cannot be converted into an integer), it throws a ValueError.
To handle this error, we use a try-except block. When the ValueError occurs, the execution jumps to the except block, and we print a message indicating that a ValueError has occurred.
This is a basic example of understanding and handling ValueError in Python. As we progress, we’ll explore more complex scenarios and advanced techniques for handling ValueErrors.
Tackling Complex Scenarios: ValueError
As you gain experience in Python, you’ll encounter more complex scenarios where a ValueError can occur. These advanced cases often involve functions or methods that expect specific input formats or certain types of values.
Consider the following example where we use the datetime
module to convert a string into a datetime object:
from datetime import datetime
def convert_to_datetime(date_string):
return datetime.strptime(date_string, '%Y-%m-%d')
try:
print(convert_to_int('2021-10-30'))
print(convert_to_int('30-10-2021'))
except ValueError as e:
print(f'Caught a ValueError: {e}')
# Output:
# 2021-10-30 00:00:00
# Caught a ValueError: time data '30-10-2021' does not match format '%Y-%m-%d'
In this example, the function convert_to_datetime
expects a string in the ‘YYYY-MM-DD’ format. It works fine when we pass ‘2021-10-30’. However, when we pass ’30-10-2021′, it raises a ValueError because the input does not match the expected format.
Again, we use a try-except block to catch and handle this error. This way, even if a ValueError occurs, our program doesn’t crash and can continue to run other tasks.
This example illustrates how ValueError can occur in more complex scenarios and how you can handle them effectively.
Exploring Alternate Error Handling Techniques
Python offers several other techniques for handling errors, such as else
and finally
clauses in try-except
blocks. These techniques provide you with greater control over your program’s flow and can make your code more readable and maintainable.
Using the else
Clause
The else
clause in a try-except
block executes when the try
block does not raise an exception. Here’s an example:
try:
num = int('123')
except ValueError:
print('A ValueError occurred!')
else:
print(f'No ValueError, the number is {num}')
# Output:
# No ValueError, the number is 123
In this example, since the try
block does not raise a ValueError, the else
block executes.
Using the finally
Clause
The finally
clause in a try-except
block executes no matter whether an exception is raised or not. It’s typically used for cleanup actions that must always be completed. Here’s an example:
try:
num = int('abc')
except ValueError:
print('A ValueError occurred!')
finally:
print('This will always execute.')
# Output:
# A ValueError occurred!
# This will always execute.
In this example, even though a ValueError occurs, the finally
block still executes.
Using else
and finally
clauses can make your error handling code more precise and easier to understand. However, they should be used judiciously as overuse can lead to code that’s harder to follow.
Troubleshooting ValueError: Avoiding Common Pitfalls
While handling ValueError in Python, there are certain common pitfalls that developers often fall into. By being aware of these, you can write more robust code and avoid unnecessary bugs.
1. Not Catching ValueError
One of the most common mistakes is simply not catching the ValueError. This can cause your program to crash unexpectedly. Always use a try-except
block when you’re dealing with operations that can potentially raise a ValueError.
# Incorrect approach
num = int('abc')
# Output:
# ValueError: invalid literal for int() with base 10: 'abc'
# Correct approach
try:
num = int('abc')
except ValueError:
print('A ValueError occurred!')
# Output:
# A ValueError occurred!
In the incorrect approach, the program crashes when it tries to convert ‘abc’ to an integer. In the correct approach, we catch the ValueError and print a message, preventing the program from crashing.
2. Overgeneralizing the Except Block
While it’s important to catch exceptions, you should avoid catching all exceptions indiscriminately. This can mask other important errors and make debugging harder.
# Incorrect approach
try:
num = int('abc')
except Exception: # catches all exceptions
print('An error occurred!')
# Correct approach
try:
num = int('abc')
except ValueError: # catches only ValueError
print('A ValueError occurred!')
In the incorrect approach, we catch all exceptions, which can hide other unrelated errors. In the correct approach, we catch only ValueError, allowing other exceptions to be raised normally.
By keeping these considerations in mind, you can handle ValueError effectively and write more reliable Python programs.
Python Exception Handling: Understanding ValueError
Python’s exception handling mechanism is a powerful tool for managing and responding to runtime errors. It’s a key part of writing robust Python code. And ValueError, as we’ve been discussing, is a part of this mechanism.
Python exceptions are organized in a hierarchy of classes, with all exceptions inheriting from the base class BaseException
. The ValueError
is a direct subclass of the Exception
class, which is itself a subclass of BaseException
.
# Hierarchy of exceptions in Python
BaseException
|
+-- Exception
|
+-- ValueError
This hierarchy is important because when you catch an exception, you also catch all of its subclasses. For example, if you catch Exception
, you’ll catch ValueError
as well, because ValueError
is a subclass of Exception
.
try:
num = int('abc')
except Exception:
print('An exception occurred!')
# Output:
# An exception occurred!
In this example, even though we’re catching Exception
and not ValueError
specifically, the ValueError is still caught because it’s a subclass of Exception
.
Understanding the hierarchy of exceptions in Python can help you write more precise error handling code and better understand the behavior of your program.
Beyond ValueError: Building Robust Python Programs
Proper error handling, including managing ValueError, is a cornerstone of creating robust Python programs. It allows your programs to respond gracefully to unexpected situations and continue running even when something goes wrong.
Exploring Related Concepts
In addition to ValueError, Python offers a variety of other built-in exceptions that you can use to handle different kinds of errors. For example, TypeError
is raised when an operation is performed on an object of an inappropriate type, and IndexError
is raised when you try to access an index that doesn’t exist in a list.
Python also allows you to define your own custom exceptions. This can be useful when you want to raise an exception that represents a specific error condition in your program.
Logging is another key aspect of building robust Python programs. By logging important events and errors in your program, you can make it easier to debug and understand what’s happening in your program.
Finally, debugging is the process of finding and fixing bugs in your program. Python provides several tools for debugging, including the built-in pdb
module.
Further Resources for Mastering Python Error Handling
To deepen your understanding of error handling in Python, here are some resources you might find helpful:
- Pytest vs. Other Python Testing Frameworks – Learn how to write clean and effective test code using Pytest’s expressive syntax and compare to othi.
How to Throw Custom Exceptions in Python – Dive into the world of exception handling and learn when and how to throw exceptions.
Handling Python Errors and Exceptions Gracefully – Discover the fundamentals of Python exceptions and how they help handle unexpected situations.
Errors and Exceptions in Python – The official tutorial from Python documentation, providing an overview on handling errors and exceptions in Python.
Logging in Python with Real Python – Real Python gives a comprehensive guide on how to utilize Python’s built-in logging capabilities.
PDB: Python’s Official Debugging Module – The official Python documentation provides detailed usage of the pdb (Python DeBugger) module for debugging Python programs.
By mastering error handling in Python, you can write more reliable, robust code and become a more effective Python developer.
Wrapping Up: Mastering ValueError in Python
In this comprehensive guide, we’ve delved into the world of ValueError in Python, a common exception that can act as a roadblock in your code execution. We’ve explored what it is, why it occurs, and how to handle it effectively to create robust Python programs.
We began with the basics, understanding the causes of ValueError and learning how to catch and handle it using try-except blocks. We then ventured into more complex scenarios, where we discussed advanced techniques for handling ValueError in Python. These techniques included using else and finally clauses in try-except blocks, which provide more control over the flow of your program.
Along the way, we addressed common pitfalls in error handling and provided solutions to avoid them. We also looked at the hierarchy of exceptions in Python, understanding how ValueError fits into it and how this hierarchy impacts your error handling code.
Method | Pros | Cons |
---|---|---|
Try-Except Block | Simple, effective, prevents program from crashing | Doesn’t specify the type of error |
Try-Except-Else Block | Executes code when no exception occurs | Can make code more complex |
Try-Except-Finally Block | Executes code regardless of whether an exception occurs | Can be overused, making code harder to follow |
Whether you’re a beginner just starting out with Python, an intermediate developer looking to level up your error handling skills, or an expert aiming to deepen your understanding of Python’s exception handling mechanism, we hope this guide has provided you with valuable insights into handling ValueError in Python.
Understanding and handling ValueError is a key part of writing reliable, robust Python code. With the knowledge you’ve gained from this guide, you’re now well-equipped to handle ValueError and write more effective Python programs. Happy coding!